Home Documentation Writing Reading Pictures1 Pictures2 Formulas Date/Time SheetByName
Merging Grouping InsertRowCol NumFormats Formats Fonts Buffer1 Buffer2 Copying TopNFilter
Sorting StringFilter NumberFilter FilterByValues Protection Replacing RichString BeginWith
ColorScale OpRule AltRows
Merging Grouping InsertRowCol NumFormats Formats Fonts Buffer1 Buffer2 Copying TopNFilter
Sorting StringFilter NumberFilter FilterByValues Protection Replacing RichString BeginWith
ColorScale OpRule AltRows
Writing a rich string with multiple fonts in one cell
This example shows how to use RichString class. See the result in the richString.xlsx file.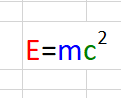
#include "libxl.h" using namespace libxl; int main() { Book* book = xlCreateXMLBook(); Sheet* sheet = book->addSheet(L"my"); RichString* rs = book->addRichString(); Font* font1 = rs->addFont(); font1->setColor(COLOR_RED); font1->setSize(24); Font* font2 = rs->addFont(); font2->setSize(24); Font* font3 = rs->addFont(); font3->setColor(COLOR_BLUE); font3->setSize(24); Font* font4 = rs->addFont(); font4->setColor(COLOR_GREEN); font4->setSize(24); Font* font5 = rs->addFont(); font5->setScript(SCRIPT_SUPER); font5->setSize(24); rs->addText(L"E", font1); rs->addText(L"=", font2); rs->addText(L"m", font3); rs->addText(L"c", font4); rs->addText(L"2", font5); sheet->writeRichStr(5, 3, rs); book->save(L"richString.xlsx"); book->release(); return 0; }